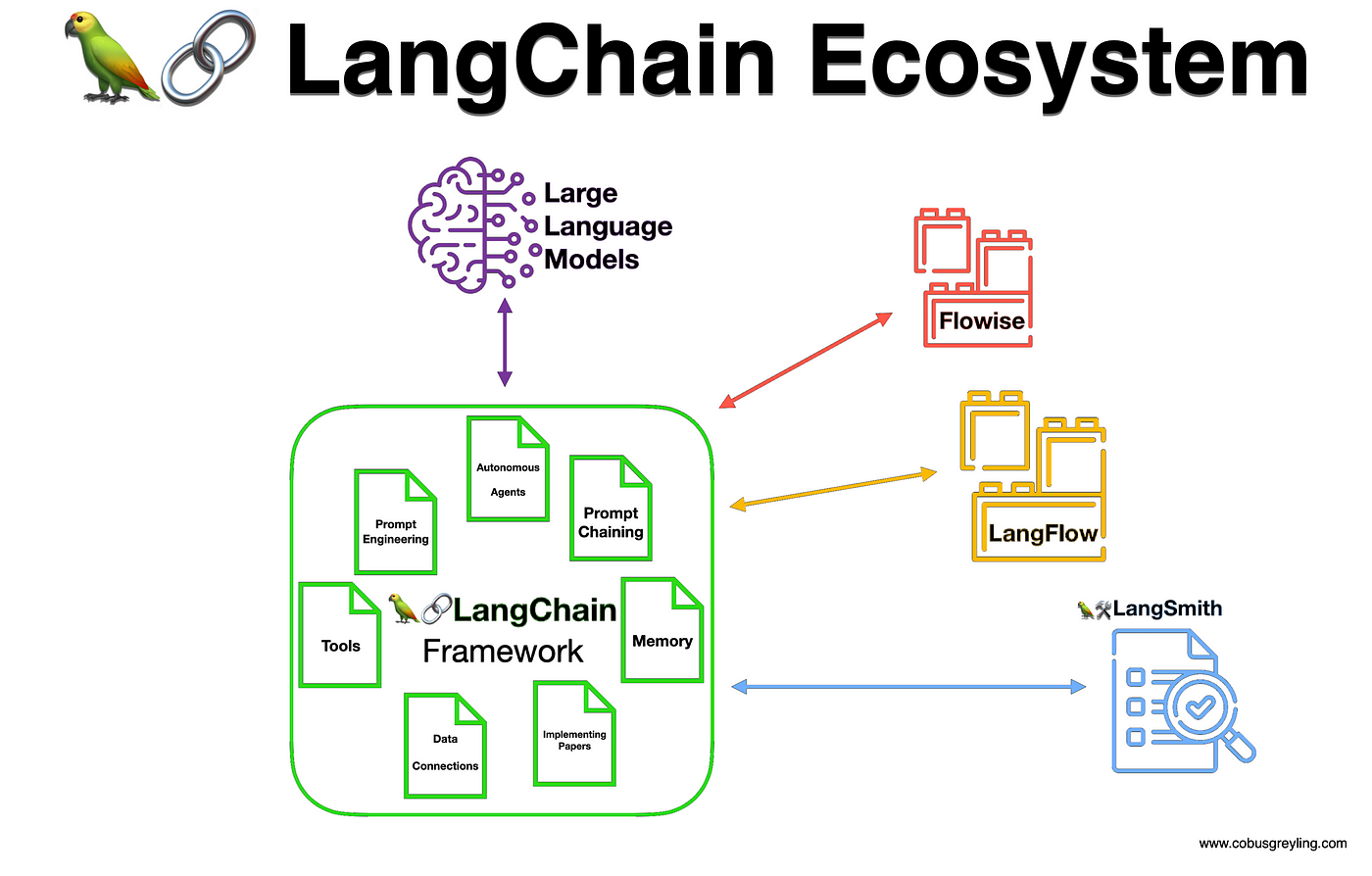
Introduction to LangChain
LangChain is an exciting new AI system that has the potential to revolutionize natural language processing. At its core, LangChain is a transformer neural network designed to have a conversational human-like dialogue. What makes LangChain unique is that it has been trained to be helpful, harmless, and honest through human feedback.
LangChain operates by taking in natural language prompts and questions from users. It then formulates thoughtful responses and can clarify or expand on its answers through continued dialogue. Unlike other AI chatbots, LangChain maintains context and memory, allowing it to hold deeper conversations. It aims to provide informative and substantiated responses, rather than speculative or untruthful information.
The goal of LangChain is not just to have an engaging conversation, but to be truly helpful for humans seeking knowledge. It strives to understand user intents and provide high-quality information to satisfy informational queries. Early testing shows LangChain has strong language generation capabilities and comprehension skills compared to other natural language AI systems.
While still an emerging technology, LangChain demonstrates the rapid progress of large language models. Its conversational nature and focus on being helpful and honest points towards a more humanistic approach in AI development. LangChain offers an exciting glimpse into how AI dialogue agents could become more beneficial knowledge resources in the future.
How LangChain Works
LangChain is powered by a hybrid system that combines both reinforcement learning and supervised learning techniques.
At a high level, LangChain utilizes a large language model that is pre-trained on massive amounts of text data. This provides the model with a strong foundation in natural language processing.
LangChain then enhances the capabilities of the language model through a reinforcement learning technique called self-play. In self-play, two instances of the model are pitted against each other in a game. One instance provides a prompt, and the other generates a response. The responses are scored, and the model is rewarded for coherent, relevant, and informative responses. Through repeated iterations of self-play, the model learns to have natural conversations.
In addition to self-play, LangChain models are also fine-tuned through supervised learning on specialized datasets. This allows them to gain skills in specific domains like customer support, coding, and more.
The hybrid reinforcement and supervised learning approach allows LangChain models to have both strong conversational abilities as well as specialized skills. The pre-training and fine-tuning process enables LangChain to produce remarkably human-like outputs across a variety of applications.
LangChain's Capabilities
LangChain has demonstrated powerful capabilities in several key areas of natural language processing:
-
Reasoning - LangChain models can follow chains of logic, make inferences, and answer questions that require reasoning skills. This sets LangChain apart from many other language models that struggle with logical reasoning.
-
Summarization - LangChain can summarize long texts into concise overviews that capture the main ideas and key details. The models are able to identify the most salient points and distill them into coherent summaries.
-
Translation - LangChain shows promise for high-quality machine translation between languages. Early results indicate LangChain may match or exceed the translation abilities of models like Google Translate. This could greatly expand access to information across language barriers.
-
Knowledge - LangChain demonstrates extensive world knowledge and common sense, likely due to its training on broad internet data. This gives the models more awareness of real-world concepts compared to narrower AI models.
-
Natural language - LangChain interacts in remarkably human-like conversational language. The models produce fluent, nuanced, and sophisticated text that approaches human levels of quality and coherence. This natural language ability drives many of LangChain's other strengths.
Overall, LangChain's versatility across multiple aspects of language processing sets it apart from previous natural language AI systems. The combination of reasoning, knowledge, summarization, translation and human-like text abilities points towards the vast possibilities of this technology going forward. LangChain represents a major step towards more general artificial intelligence.
Chatbot Example
Here's a simple example of how to write a multiple PDF chatbot with LangChain:
import streamlit as st
from dotenv import load_dotenv
from PyPDF2 import PdfReader
from langchain.text_splitter import CharacterTextSplitter
from langchain.embeddings import OpenAIEmbeddings,
HuggingFaceInstructEmbeddings
from langchain.vectorstores import FAISS
from langchain.memory import ConversationBufferMemory
from langchain.chains import ConversationalRetrievalChain
from langchain.chat_models import ChatOpenAI
from htmlTemplates import bot_template, user_template, css
from transformers import pipeline
def get_pdf_text(pdf_files):
text = ""
for pdf_file in pdf_files:
reader = PdfReader(pdf_file)
for page in reader.pages:
text += page.extract_text()
return text
def get_chunk_text(text):
text_splitter = CharacterTextSplitter(
separator = "\n",
chunk_size = 1000,
chunk_overlap = 200,
length_function = len
)
chunks = text_splitter.split_text(text)
return chunks
def get_vector_store(text_chunks):
# For OpenAI Embeddings
embeddings = OpenAIEmbeddings()
# For Huggingface Embeddings
vectorstore = FAISS.from_texts(texts = text_chunks,
embedding = embeddings)
return vectorstore
def get_conversation_chain(vector_store):
# OpenAI Model
llm = ChatOpenAI()
# HuggingFace Model
# llm = HuggingFaceHub(repo_id="google/flan-t5-xxl",
model_kwargs={"temperature":0.5,
"max_length":512})
memory = ConversationBufferMemory(memory_key='chat_history',
return_messages=True)
conversation_chain = ConversationalRetrievalChain.from_llm(
llm = llm,
retriever = vector_store.as_retriever(),
memory = memory
)
return conversation_chain
def handle_user_input(question):
response = st.session_state.conversation({'question':question})
st.session_state.chat_history = response['chat_history']
for i, message in enumerate(st.session_state.chat_history):
if i % 2 == 0:
st.write(user_template.replace("{{MSG}}",
message.content),
unsafe_allow_html=True)
else:
st.write(bot_template.replace("{{MSG}}",
message.content),
unsafe_allow_html=True)
def main():
load_dotenv()
st.set_page_config(page_title='Chat with Your own PDFs',
page_icon=':books:')
st.write(css, unsafe_allow_html=True)
if "conversation" not in st.session_state:
st.session_state.conversation = None
if "chat_history" not in st.session_state:
st.session_state.chat_history = None
st.header('Chat with Your own PDFs :books:')
question = st.text_input("Ask anything to your PDF: ")
if question:
handle_user_input(question)
with st.sidebar:
st.subheader("Upload your Documents Here: ")
pdf_files = st.file_uploader("Choose your PDF Files and Press OK",
type=['pdf'], accept_multiple_files=True)
if st.button("OK"):
with st.spinner("Processing your PDFs..."):
# Get PDF Text
raw_text = get_pdf_text(pdf_files)
# Get Text Chunks
text_chunks = get_chunk_text(raw_text)
# Create Vector Store
vector_store = get_vector_store(text_chunks)
st.write("DONE")
# Create conversation chain
st.session_state.conversation = get_conversation_chain(vector_store)
if __name__ == '__main__':
main()
import streamlit as st
from dotenv import load_dotenv
from PyPDF2 import PdfReader
from langchain.text_splitter import CharacterTextSplitter
from langchain.embeddings import OpenAIEmbeddings,
HuggingFaceInstructEmbeddings
from langchain.vectorstores import FAISS
from langchain.memory import ConversationBufferMemory
from langchain.chains import ConversationalRetrievalChain
from langchain.chat_models import ChatOpenAI
from htmlTemplates import bot_template, user_template, css
from transformers import pipeline
def get_pdf_text(pdf_files):
text = ""
for pdf_file in pdf_files:
reader = PdfReader(pdf_file)
for page in reader.pages:
text += page.extract_text()
return text
def get_chunk_text(text):
text_splitter = CharacterTextSplitter(
separator = "\n",
chunk_size = 1000,
chunk_overlap = 200,
length_function = len
)
chunks = text_splitter.split_text(text)
return chunks
def get_vector_store(text_chunks):
# For OpenAI Embeddings
embeddings = OpenAIEmbeddings()
# For Huggingface Embeddings
vectorstore = FAISS.from_texts(texts = text_chunks,
embedding = embeddings)
return vectorstore
def get_conversation_chain(vector_store):
# OpenAI Model
llm = ChatOpenAI()
# HuggingFace Model
# llm = HuggingFaceHub(repo_id="google/flan-t5-xxl",
model_kwargs={"temperature":0.5,
"max_length":512})
memory = ConversationBufferMemory(memory_key='chat_history',
return_messages=True)
conversation_chain = ConversationalRetrievalChain.from_llm(
llm = llm,
retriever = vector_store.as_retriever(),
memory = memory
)
return conversation_chain
def handle_user_input(question):
response = st.session_state.conversation({'question':question})
st.session_state.chat_history = response['chat_history']
for i, message in enumerate(st.session_state.chat_history):
if i % 2 == 0:
st.write(user_template.replace("{{MSG}}",
message.content),
unsafe_allow_html=True)
else:
st.write(bot_template.replace("{{MSG}}",
message.content),
unsafe_allow_html=True)
def main():
load_dotenv()
st.set_page_config(page_title='Chat with Your own PDFs',
page_icon=':books:')
st.write(css, unsafe_allow_html=True)
if "conversation" not in st.session_state:
st.session_state.conversation = None
if "chat_history" not in st.session_state:
st.session_state.chat_history = None
st.header('Chat with Your own PDFs :books:')
question = st.text_input("Ask anything to your PDF: ")
if question:
handle_user_input(question)
with st.sidebar:
st.subheader("Upload your Documents Here: ")
pdf_files = st.file_uploader("Choose your PDF Files and Press OK",
type=['pdf'], accept_multiple_files=True)
if st.button("OK"):
with st.spinner("Processing your PDFs..."):
# Get PDF Text
raw_text = get_pdf_text(pdf_files)
# Get Text Chunks
text_chunks = get_chunk_text(raw_text)
# Create Vector Store
vector_store = get_vector_store(text_chunks)
st.write("DONE")
# Create conversation chain
st.session_state.conversation = get_conversation_chain(vector_store)
if __name__ == '__main__':
main()
Comparing LangChain to Other Models
LangChain builds on previous large language models like GPT-3, PaLM, and others, but has some key differences that set it apart:
-
While GPT-3 and PaLM are "generalist" models trained on huge datasets of internet text, LangChain is trained more narrowly on human conversations. This makes LangChain better at natural dialogue.
-
LangChain incorporates reinforcement learning, allowing it to get feedback during conversations on whether responses are sensible and on-topic. This helps improve conversational ability over time.
-
LangChain uses a technique called chain-of-thought prompting to have more contextual awareness in ongoing conversations. The model pays attention to what was discussed previously in the conversation when formulating new responses.
-
LangChain has substantially fewer parameters than models like GPT-3 and PaLM with trillions of parameters. LangChain has about 760 million parameters, making it more lightweight and usable.
-
Anthropic, the creators of LangChain, claim their model has better common sense, social awareness, and harmless intent compared to predecessors. Safety was a priority in LangChain's training.
-
LangChain is designed to be helpful, harmless, and honest when deployed in actual applications. Anthropic has mechanisms in place to monitor behavior and pull the plug on LangChain if harmful outputs emerge.
So in summary, LangChain advances the state of the art in dialog agents through specialized training on human conversations, reinforcement learning, chained prompting, and an emphasis on safety. This helps it hold more natural and coherent conversations compared to previous large language models.
LangChain Use Cases
LangChain is currently in the early stages of research and development, but it has exciting potential for many real-world applications if further developed. Here are some possible use cases:
-
Natural language processing: LangChain could be used to build more human-like chatbots, voice assistants, and other AI systems that can engage in nuanced conversations. Its capabilities in understanding context and generating coherent responses could significantly advance natural language AI.
-
Content generation: LangChain shows promise for automating content writing for things like news articles, reports, stories, and more. It may one day be able to generate high-quality, original content tailored to specific topics and styles.
-
Creative writing: LangChain's conversational abilities could make it useful as a creative writing aid. It could collaborate with human writers, propose ideas, expand outlines, generate draft passages, and refine wording and style.
-
Education and tutoring: LangChain could potentially tutor students by answering questions in different subjects, explaining concepts in its own words, and providing feedback on assignments. Its conversational nature could make it an interactive teaching tool.
-
Personal assistance: LangChain's conversational capabilities could power next-generation personal assistants that not only complete tasks but also offer companionship through natural chats.
-
Accessibility: LangChain could be leveraged to improve accessibility for people with disabilities through automatically generated captions, reading text aloud, summarizing content, and translating languages.
The open-ended nature of LangChain means there are likely many more applications that have yet to be envisioned. As the technology improves, LangChain could become a versatile AI assistant for diverse tasks involving language and communication.
Current Limitations of LangChain
LangChain is an exciting new AI system, but it still has some limitations compared to human intelligence:
-
Lack of common sense - Like other AI systems, LangChain lacks the common sense, intuition and general knowledge that humans accumulate through life experience. This can lead to nonsensical or illogical responses.
-
Inability to verify facts - LangChain cannot independently verify facts or sources of information. It relies on its training data, so any biases or errors in that data get propagated.
-
Narrow expertise - While good at certain tasks, LangChain does not have broad general intelligence. Its knowledge is constrained to what it has been trained on, and lacks adaptability.
-
Brittleness - Small mistakes or misunderstandings can lead to nonsensical outputs. LangChain's responses break down more easily compared to the robustness of human understanding.
-
Lack of creativity - LangChain cannot engage in highly creative work like storytelling, humor or poetry generation. It is focused on straightforward information tasks.
-
No sense of self - Unlike humans, LangChain has no inner mental life or concept of self. This limits its ability to deeply understand the human experience.
While powerful, LangChain is not yet comparable to human-level AI. It has excellent capabilities within its training domain, but still has clear limitations compared to flexible, creative, and broadly intelligent human beings. Overcoming these limitations remains a grand challenge for AI researchers.
The Future of LangChain
LangChain is still in its early stages, but shows great promise as an AI technique for natural language tasks. As research continues, we can expect to see improvements in several areas:
-
Increased capabilities and accuracy - The underlying AI models that power LangChain will continue to be refined, allowing for more complex conversational abilities, higher accuracy in responding to prompts, and handling more nuanced or subjective topics.
-
Broader knowledge - Right now, LangChain has a limited knowledge base to draw on. Expanding the information it can access, such as through integrating various databases and corpora, will make its responses more knowledgeable and useful.
-
Specialized applications - Rather than being a general conversational AI, LangChain models tailored for specific domains like customer service, technical support, or medical diagnosis could be developed. This would allow for very sophisticated domain-specific applications.
-
Multimodal applications - Current LangChain models operate solely with text. Enhancing them to integrate and understand other modes like images, audio and video could greatly expand their capabilities.
-
Efficiency improvements - Reducing the computational resources required for training and running LangChain models would make the technology more accessible and scalable. Compression techniques are one avenue being explored.
-
Ethical safeguards - As capabilities improve, ethical risks like bias and misinformation will need to be proactively addressed through techniques like human oversight, bias testing, and improved model transparency.
The pace of progress in AI means we likely can't foresee everything LangChain will enable in the future. But continued research seems certain to unlock new frontiers in human-computer interaction and natural language processing.
Controversies and Ethical Considerations
As with any powerful new technology, LangChain comes with risks as well as benefits. While the capabilities of large language models like LangChain are impressive, concerns have been raised about potential misuse of the technology.
One area of concern is the generation of harmful, biased, or misleading content. While LangChain's creators at Anthropic have implemented safety measures to mitigate these risks, the AI still has the potential to generate problematic text if used irresponsibly. There are fears that LangChain could be used to automate the spread of misinformation, harassment, or extremist content at scale.
Another consideration is the impact on creative professionals whose work may be replicated or replaced by AI systems like LangChain. Some view this technology as a threat to human creativity and livelihoods. There are concerns about copyright, attribution, and proper acknowledgement when AI is used to generate written works.
The impressive abilities demonstrated by LangChain also raise concerns about how authentic human-generated content can be differentiated from AI output. Tools to detect machine-generated text exist, but constantly evolving AI could challenge efforts to discern real vs artificial content. Some argue the technology should always disclose when text is AI-generated to avoid deception.
As with any transformative technology, norms, regulations and safeguards may be needed to steer the development of large language models like LangChain toward the greatest societal benefit. With an open and thoughtful approach, experts hope AI like this can empower human creativity while mitigating risks. But ethical questions surrounding the appropriate and safe deployment of systems like LangChain remain up for debate.
Expert Perspectives on LangChain
LangChain has generated a lot of discussion and commentary among experts in artificial intelligence, linguistics, and related fields. Here are some notable perspectives:
-
AI researcher Anthropic has argued that large language models like LangChain need to be developed responsibly, with a focus on safety and ethics. There are concerns about potential harms if systems become too capable and uncontrolled.
-
Linguist Emily Bender thinks LangChain highlights issues with how language models are built, often scraping data without concern for copyright or ethics. She argues technologists should work more closely with linguists.
-
Google AI researcher Blaise Aguera y Arcas has said LangChain represents important progress in conversational AI. However, we need to be cautious about claims of human-level intelligence, which remains beyond current technology.
-
Philosopher David Chalmers believes chatbots like LangChain force us to think more deeply about consciousness. We should not assume advanced conversational ability implies a conscious experience like humans have.
-
Facebook VP Jérôme Pesenti cautions that while LangChain is impressive, it still makes factual mistakes and lacks robust reasoning abilities. Significant research is needed before AI like this can be deployed safely.
-
MIT professor Max Tegmark argues LangChain provides further evidence that AI safety research needs to accelerate to keep pace with rapid technical improvements. We cannot wait to establish guardrails for this technology.
Conclusion
LangChain is an exciting new AI system that has the potential to revolutionize natural language processing. This article provided an overview of how LangChain works, its current capabilities, limitations, and future potential.
In summary, LangChain demonstrates an AI's ability to have a conversational "chain of thought", maintain context and memory, and respond to follow-up questions and challenges. This more closely mimics human conversation. While still early in development, LangChain shows promise for use cases like search, dialogue agents, and content generation.
However, there are valid concerns around bias, safety, and misuse of the technology that developers need to consider. Powerful language models like LangChain should be handled responsibly. More research is needed to improve the robustness and alignment of such AI systems.
Overall, LangChain represents an impressive step forward in conversational AI. While the technology is not perfect, it provides an exciting glimpse into more human-like language abilities in machines. Responsible development and rigorous testing will be critical as LangChain and similar models continue to evolve. If cultivated properly, these large language models could enable incredibly useful applications while minimizing risks. The future looks bright, but we must guide it wisely.